Flutterでアプリ下部にナビゲーションメニューを設置するサンプル
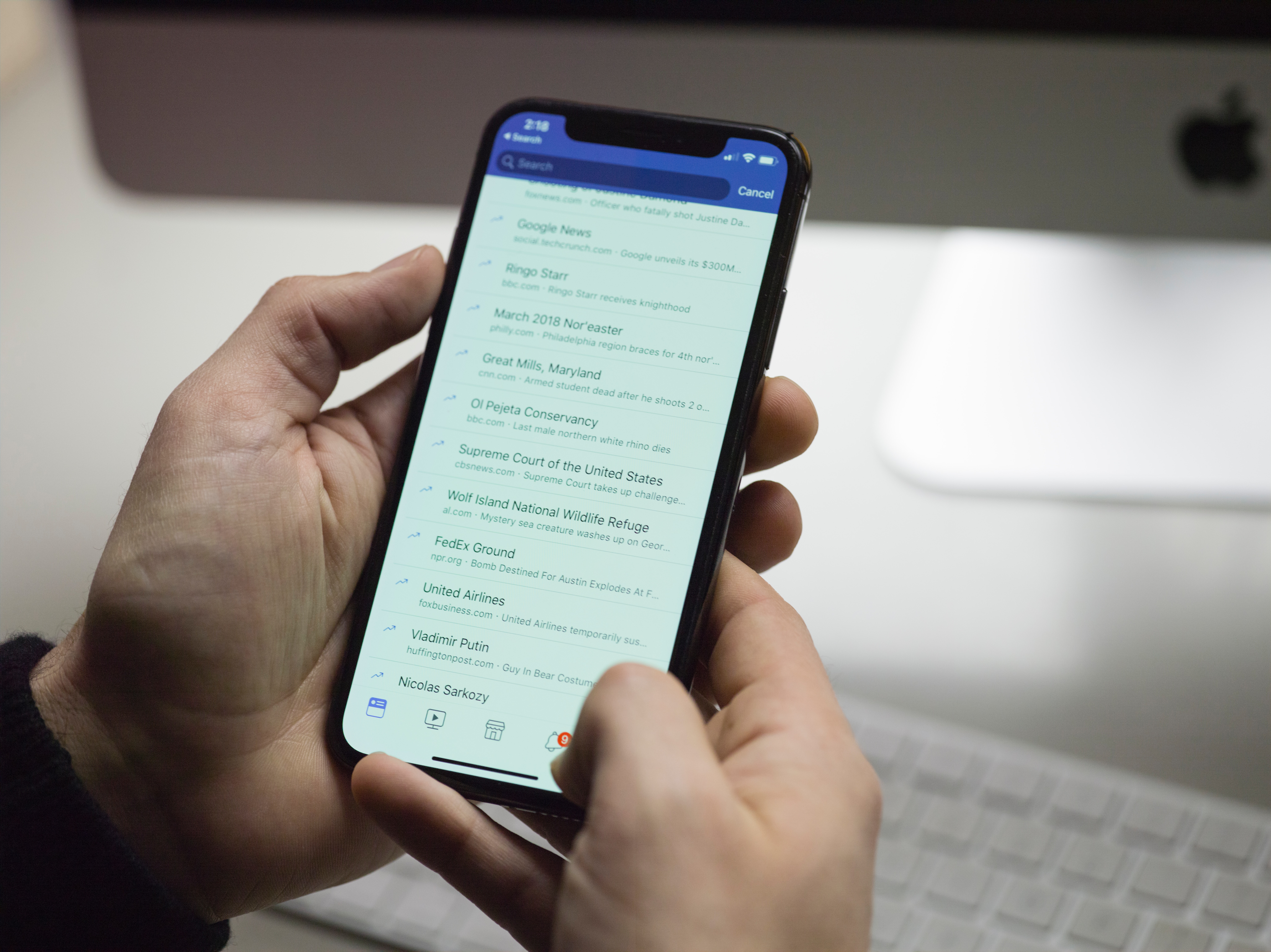
はじめに
色々なスマホアプリでページ下部にメニューがあり、タップすると画面が切り替わる機能ががあります。ボトムナビゲーションメニュー等といい、Flutterでは、BottomNavigationBar
とPageView
を使うことで簡単に実装できます。この記事では、これらを使った簡単な例をまとめます。
できるようになること
以下のGIF画像のように、アプリ下部にナビゲーションメニューがあり、タップしたり水平方向にページをスワイプすることでページを切り替えることができます。
デモのサンプルコード
上記のGIF画像のデモのサンプルコードを以下に載せます。以下のコードの元はflutter create
で作成したカウンターアプリです。BottomNavigationBar
とPageView
に関連する部分にはコメントを記載しています。
main.dartのコード
まずはじめにmain.dart
です。
SingleTickerProviderStateMixin
を使用していますが、これについては以下が大変参考になりました。
また、ページ切替時のアニメーションの挙動については、animateToPage
のオプションで指定しています。Duration
で遷移にかける時間、curve
で挙動を指定できます。このcurve
については、以下の公式ドキュメントにどのようなものを指定できてそれぞれどのような挙動かまとまっています。
ナビゲーションメニューに対応するビューのコード
あとは、アプリ下部のナビゲーションメニューに対応するページビューを3つ用意します。ここでは、適当にpages
という名前のディレクトリをlib
配下に作成し、そこにpages/BookScreen.dart
、pages/CloudScreen.dart
、pages/CakeScreen.dart
を作成しました。なお、これら3つはほぼ全て同じ内容で色やアイコンを変更しているだけです。
以下はpages/CloudScreen.dart
です。
以下はpages/CakeScreen.dart
です。
まとめ
アプリ下部に表示する基本的なナビゲーションメニューを実装しました。
関連記事
- 公開日:2022/08/30 更新日:2022/08/30
FlutterアプリでAndroid StudioのGenerate Signed Bundle/APKが表示されない時の対処法
FlutterアプリをGoogle Play Storeに公開するためにAndroid Studioを使ってアップロード鍵を生成しようとしたところ、公式ドキュメントに書かれている「Build」→「Generate Signed Bundle/APK」というメニューが見つかりませんでした。この解決法をメモします。
- 公開日:2022/08/15 更新日:2022/08/15
webview_flutterを使ってFlutterアプリ内でWebページを開く
FlutterでURLをタップした時にアプリ内でそのURLのWebページを開く方法についてまとめます。この記事では、Flutter公式のプラグインであるwebview_flutterを使用した実装例をメモします。
- 公開日:2022/08/14 更新日:2022/08/14
FlutterでURLへのリンクを作成してアプリ外でWebページを開く
FlutterでURLをタップしたらブラウザが開いてそこでURL先を表示したい場合があります。この記事では、Flutter公式のプラグインを使用した実装例をメモします。
- 公開日:2019/12/03 更新日:2019/12/03
Android App Bundleを実機にインストールして試すために使うbundletoolの使い方
Flutterなどで開発したAndroidアプリを自分の手元にある実機にインストールして試したい場合はbundletoolを使用するよう公式ドキュメントに記載されています。ただ、常識であるためなのか詳しい使い方が書かれておらず戸惑ったのでメモしておきます。
- 公開日:2019/12/02 更新日:2019/12/02
Flutterでアプリの復帰やサスペンドを検出して処理を実行する
Flutterで開発したアプリが復帰した時やサスペンドした時を検出して任意の処理を実行するための手順をまとめます。
開発アプリ
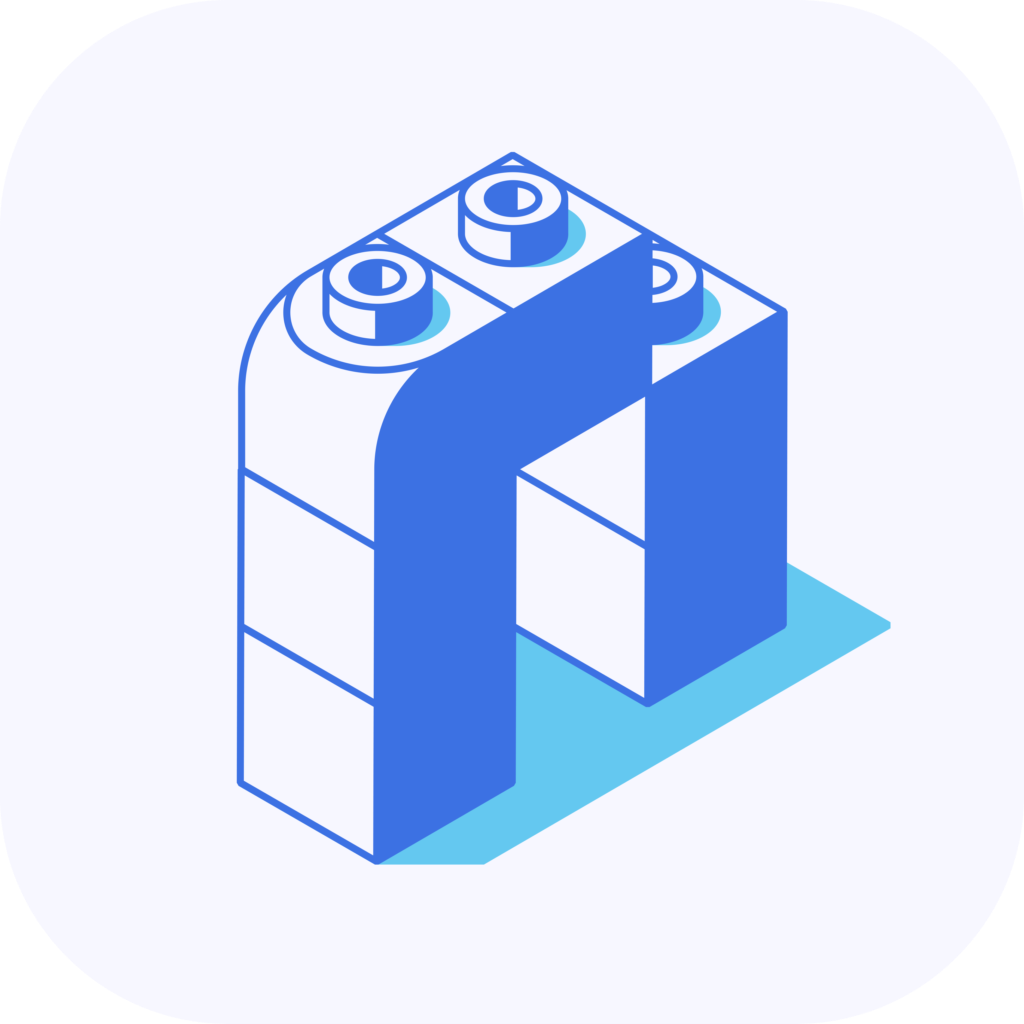